Overview
This Flask application is a simple user authentication system using Flask, Flask-WTF, WTForms, MySQL, and bcrypt for password hashing. The application allows users to register, log in, and access a dashboard page after successful login.
Features:
- User Registration: Allows users to sign up with their name, email, and password.
- Login: Users can log in with their email and password.
- Dashboard: Authenticated users can access a personal dashboard.
- Logout: Users can log out and end their session.
Requirements:
Before running the application, you need to install the necessary dependencies.
- Install Python 3.x (if not already installed).
- Installing and setting Flask.
- python -m venv vevn
- .\venv\Scripts\activate (these is to activate your virtual environment, if error occur you can manually open them usind cd command in the terminal)
- Select python interpretor (if you are using VScose à select view à command palette à python select interpreter à enter interpreter path à. à \your_project\venv\Scripts\python.exe)
- python -m pip install –upgrade pip (if needed to upgrade)
- python -m pip install flask (to install flask)
- create new app.py
- python -m flask –app .\app.py run OR python -m flask run (for running the flask application)
- Install the following packages using pip:
- pip install Flask
- pip install Flask-WTF
- pip install flask-mysqldb
- pip install bcrypt
Ensure that MySQL is installed and running on your local machine, and you have to created a database called mydatabase with a table users containing the following fields:
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100) UNIQUE,
password VARCHAR(255));
Application Structure
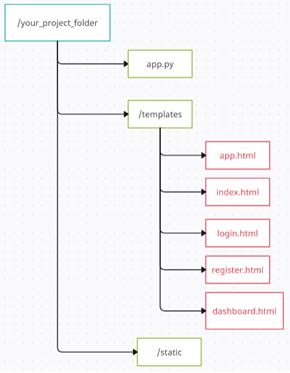
app.py
This is the main Python file that contains the Flask application and route definitions.
These file contains the below code
from flask import Flask, render_template, redirect, url_for, session, flash
from flask_wtf import FlaskForm
from wtforms import StringField,PasswordField,SubmitField
from wtforms.validators import DataRequired, Email, ValidationError
import bcrypt
from flask_mysqldb import MySQL
app = Flask(__name__)
# MySQL Configuration
app.config['MYSQL_HOST'] = 'localhost'
app.config['MYSQL_USER'] = 'root'
app.config['MYSQL_PASSWORD'] = ''
app.config['MYSQL_DB'] = 'mydatabase'
app.secret_key = 'your_secret_key_here'
mysql = MySQL(app)
class RegisterForm(FlaskForm):
name = StringField("Name",validators=[DataRequired()])
email = StringField("Email",validators=[DataRequired(), Email()])
password = PasswordField("Password",validators=[DataRequired()])
submit = SubmitField("Register")
def validate_email(self,field):
cursor = mysql.connection.cursor()
cursor.execute("SELECT * FROM users where email=%s",(field.data,))
user = cursor.fetchone()
cursor.close()
if user:
raise ValidationError('Email Already Taken')
class LoginForm(FlaskForm):
email = StringField("Email",validators=[DataRequired(), Email()])
password = PasswordField("Password",validators=[DataRequired()])
submit = SubmitField("Login")
@app.route('/')
def index():
return render_template('index.html')
@app.route('/register',methods=['GET','POST'])
def register():
form = RegisterForm()
if form.validate_on_submit():
name = form.name.data
email = form.email.data
password = form.password.data
hashed_password = bcrypt.hashpw(password.encode('utf-8'),bcrypt.gensalt())
# store data into database
cursor = mysql.connection.cursor()
cursor.execute("INSERT INTO users (name,email,password) VALUES (%s,%s,%s)",(name,email,hashed_password))
mysql.connection.commit()
cursor.close()
return redirect(url_for('login'))
return render_template('register.html',form=form)
@app.route('/login',methods=['GET','POST'])
def login():
form = LoginForm()
if form.validate_on_submit():
email = form.email.data
password = form.password.data
cursor = mysql.connection.cursor()
cursor.execute("SELECT * FROM users WHERE email=%s",(email,))
user = cursor.fetchone()
cursor.close()
if user and bcrypt.checkpw(password.encode('utf-8'), user[3].encode('utf-8')):
session['user_id'] = user[0]
return redirect(url_for('dashboard'))
else:
flash("Login failed. Please check your email and password")
return redirect(url_for('login'))
return render_template('login.html',form=form)
@app.route('/dashboard')
def dashboard():
if 'user_id' in session:
user_id = session['user_id']
cursor = mysql.connection.cursor()
cursor.execute("SELECT * FROM users where id=%s",(user_id,))
user = cursor.fetchone()
cursor.close()
if user:
return render_template('dashboard.html',user=user)
return redirect(url_for('login'))
@app.route('/logout')
def logout():
session.pop('user_id', None)
flash("You have been logged out successfully.")
return redirect(url_for('login'))
if __name__ == '__main__':
app.run(debug=True)
Detailed Explanation for above code-
1. Flask Configuration
app = Flask(__name__)
# MySQL Configuration
app.config['MYSQL_HOST'] = 'localhost'
app.config['MYSQL_USER'] = 'root'
app.config['MYSQL_PASSWORD'] = ''
app.config['MYSQL_DB'] = 'mydatabase'
app.secret_key = 'your_secret_key_here'
mysql = MySQL(app)
- Flask Configuration: Initializes a Flask application with a secret key used for session management.
- MySQL Configuration: Configures the app to connect to a MySQL database (mydatabase) using the flask_mysqldb extension.
2. User Registration Form (RegisterForm)
class RegisterForm(FlaskForm):
name = StringField("Name", validators=[DataRequired()])
email = StringField("Email", validators=[DataRequired(), Email()])
password = PasswordField("Password", validators=[DataRequired()])
submit = SubmitField("Register")
def validate_email(self, field):
cursor = mysql.connection.cursor()
cursor.execute("SELECT * FROM users where email=%s", (field.data,))
user = cursor.fetchone()
cursor.close()
if user:
raise ValidationError('Email Already Taken')
- Form Fields:
- name: Required field for the user’s name.
- email: Required field for the user’s email with email validation.
- password: Required field for the user’s password.
- submit: Submit button to submit the registration form.
- Email Validation: Checks if the provided email already exists in the database. If the email is taken, a validation error is raised.
3. User Login Form (LoginForm)
class LoginForm(FlaskForm):
email = StringField("Email", validators=[DataRequired(), Email()])
password = PasswordField("Password", validators=[DataRequired()])
submit = SubmitField("Login")
Form Fields:
- email: Required field for the user’s email with email validation.
- password: Required field for the user’s password.
- submit: Submit button to submit the login form.
4. Routes
4.1. Home Route (/)
@app.route('/')
def index():
return render_template('index.html')
Form Fields:
- Purpose: Renders the home page (index.html), which can be a simple welcome page or landing page.
4.2. Registration Route (/register)
@app.route('/register', methods=['GET', 'POST'])
def register():
form = RegisterForm()
if form.validate_on_submit():
name = form.name.data
email = form.email.data
password = form.password.data
# Hash password
hashed_password = bcrypt.hashpw(password.encode('utf-8'), bcrypt.gensalt())
# Store data in MySQL
cursor = mysql.connection.cursor()
cursor.execute("INSERT INTO users (name, email, password) VALUES (%s, %s, %s)", (name, email, hashed_password))
mysql.connection.commit()
cursor.close()
return redirect(url_for('login'))
return render_template('register.html', form=form)
Form Fields:
- Purpose: Renders the registration form. On successful form submission, it hashes the password using bcrypt and stores the user’s name, email, and hashed password in the users table in the MySQL database.
- Redirect: After successful registration, it redirects to the login page.
4.3. Login Route (/login)
@app.route('/login', methods=['GET', 'POST'])
def login():
form = LoginForm()
if form.validate_on_submit():
email = form.email.data
password = form.password.data
# Fetch user from database
cursor = mysql.connection.cursor()
cursor.execute("SELECT * FROM users WHERE email=%s", (email,))
user = cursor.fetchone()
cursor.close()
# Check if user exists and password matches
if user and bcrypt.checkpw(password.encode('utf-8'), user[3].encode('utf-8')):
session['user_id'] = user[0]
return redirect(url_for('dashboard'))
else:
flash("Login failed. Please check your email and password")
return redirect(url_for('login'))
return render_template('login.html', form=form)
- Purpose: Renders the login form. On successful form submission, it checks if the user exists and if the provided password matches the hashed password stored in the database.
- Session Management: If login is successful, the user’s ID is stored in the session, and they are redirected to the dashboard page.
4.4. Dashboard Route (/dashboard)
@app.route('/dashboard')
def dashboard():
if 'user_id' in session:
user_id = session['user_id']
cursor = mysql.connection.cursor()
cursor.execute("SELECT * FROM users WHERE id=%s", (user_id,))
user = cursor.fetchone()
cursor.close()
if user:
return render_template('dashboard.html', user=user)
return redirect(url_for('login'))
Purpose: Displays the dashboard for authenticated users. If the user is not logged in (i.e., user_id not in session), they are redirected to the login page.
4.5. Logout Route (/logout)
@app.route('/logout')
def logout():
session.pop('user_id', None)
flash("You have been logged out successfully.")
return redirect(url_for('login'))
Purpose: Logs out the user by removing their user_id from the session and then redirects them to the login page with a flash message indicating successful logout.
Running the Application
To run the application, execute the following command in your terminal:
python app.py
This will start a local development server, and you can visit the application in your browser at :
http://127.0.0.1:5000/
Notes
- Password Hashing: The passwords are securely hashed using bcrypt before being stored in the database. This ensures that plain-text passwords are not stored.
- Session Management: The session object is used to manage user authentication. When a user logs in, their user_id is saved in the session. On logout, the session is cleared.
\templates-
The templates in this Flask application provide the structure for the user interface, using Bootstrap for styling and Flask-WTF for handling forms and validation. The app.html template serves as the base layout, while the other templates (index.html, login.html, register.html, and dashboard.html) extend it to display specific content for the home page, login, registration, and user dashboard respectively. Flash messages and form validation are included for better user experience and interaction.
1. app.html – Base Template
File Location: /templates/app.html
Purpose:
This is the base HTML layout that other templates will extend. It contains the common structure and includes external resources like Bootstrap for styling, and jQuery and Popper.js for Bootstrap’s interactive features. The {% block content %} is used as a placeholder where child templates can inject their specific content.
Structure:
Flask MySQL
{% block content %}
{% endblock %}
Key Points:
- Bootstrap Integration: Bootstrap’s CSS and JS are included through CDN links for responsive layout and UI components.
- {% block content %}: This is where the content from child templates will be inserted.
- Reusable Structure: Other templates can extend this base and only focus on the unique content for each page.
2. dashboard.html – Dashboard Page
File Location: /templates/dashboard.html
Purpose:
This template is used to display the user’s dashboard after a successful login. It provides the user’s information (like name and email) and includes a “Logout” button. If the user is not logged in, the page will not render the user information.
Structure:
{% extends 'app.html' %}
{% block content %}
Welcome to the Dashboard
{% if user %}
User Information:
- Name: {{ user[1] }}
- Email: {{ user[2] }}
Logout
{% endif %}
{% endblock %}
Key Points:
- Extends app.html: Inherits the layout and structure from app.html.
- Conditional Rendering: The user information is only displayed if a valid user object is passed to the template.
- User Details: The user’s name and email are accessed via user[1] and user[2] respectively (assuming the query returns the user in a tuple with id, name, and email).
- Logout Link: Provides a button that logs the user out by redirecting them to /logout.
3. index.html – Home Page
File Location: /templates/index.html
Purpose:
This template represents the home page of the application. It provides links to navigate to the login page and possibly other parts of the app.
Structure:
{% extends 'app.html' %}
{% block content %}
Home Page
Go to Login Page
{% endblock %}
Key Points:
- Extends app.html: Inherits the common layout from the base template.
- Simple Welcome Page: Displays a heading and a link to the login page (/login).
- Navigation: Offers a straightforward navigation path for users who have not logged in yet.
4. login.html – Login Form Page
File Location: /templates/login.html
Purpose:
This template contains the login form. It allows users to log in by providing their email and password. It also handles form validation errors and displays any flashed messages (like invalid credentials or form errors).
Structure:
{% extends 'app.html' %}
{% block content %}
Login Form
{% with messages = get_flashed_messages() %}
{% if messages %}
{% for message in messages %}
- {{ message }}
{% endfor %}
{% endif %}
{% endwith %}
{% endblock %}
Key Points:
- Flash Messages: Displays any error messages (like invalid email or password) using Flask’s get_flashed_messages() function.
- Form Handling: The form is rendered using WTForms and includes proper validation. Errors are shown next to each form field if they occur.
- Form Submission: On successful form submission, the form posts back to /login for authentication.
5. register.html – Registration Form Page
File Location: /templates/register.html
Purpose:
This template provides the registration form where users can sign up by entering their name, email, and password. Like the login form, this template also includes validation and error handling.
{% extends 'app.html' %}
{% block content %}
Register Form
{% endblock %}
Key Points:
- Form Fields: Similar to the login form, this template includes fields for name, email, and password.
- Error Handling: If any validation errors occur (such as invalid email or password format), they are displayed below the corresponding input fields.
- Form Submission: The form submits to /register for processing.
General Notes for All Templates:
- Bootstrap Classes: All templates utilize Bootstrap’s grid system and utility classes for styling and responsive design.
- form.hidden_tag(): This is used to include any hidden CSRF token required for form submissions, as part of Flask-WTF security.
- Messages & Error Handling: Flash messages and validation errors are displayed to ensure a good user experience.